Vb.net Public Private Key Generator
Authored by: Rackspace SupportOne effective way of securing SSH access to your cloud server is to usea public-private key pair. Generate RSA keys with SSH by using PuTTYgen. Generate ssh key putty and use in centos. Last updated on: 2016-06-23.
- Vb.net Public Private Key Generator Software
- Vb.net Public Private Key Generator For Bitcoin And Ethereum
- Public Private Key Encryption
- Vb.net Public Private Key Generator 2018
- Vb.net Public Private Key Generator V2 4 Full Version
- Vb.net Public Private Key Generator Blockchain
In order to be able to create a digital signature, you need a private key. (Its corresponding public key will be needed in order to verify the authenticity of the signature.)
In some cases the key pair (private key and corresponding public key) are already available in files. In that case the program can import and use the private key for signing, as shown in Weaknesses and Alternatives.
- VB.NET How do you get the private key? Usually, you generate it yourself, either using Rebex KeyGenerator sample, our key-generator API or a third-party utility (most SSH/SFTP vendors provide one). In case you already have your private key, just load it into the SshPrivateKey object - it supports lot of private key formats.
- Generate RSA pgp key with C# and VB.NET. RSA'private key password'compressionhashingcypher) ' Now we can use the key from the KeyStore or export it End Sub End Class The above code generates a key pair that does not expire. Usually, we will send the public key part of it to our partners.
8.2 Using PuTTYgen, the PuTTY key generator. PuTTYgen is a key generator. It generates pairs of public and private keys to be used with PuTTY, PSCP, and Plink, as well as the PuTTY authentication agent, Pageant (see chapter 9). PuTTYgen generates RSA, DSA, ECDSA, and Ed25519 keys. Apr 29, 2018 Bitcoin Private Key Generator 2019 Working 100% Link download. Programming in Visual Basic.Net How to Connect Access Database to VB.Net - Duration: 19:11. IBasskung 25,351,055 views.
In other cases the program needs to generate the key pair. A key pair is generated by using the KeyPairGenerator
class.
In this example you will generate a public/private key pair for the Digital Signature Algorithm (DSA). You will generate keys with a 1024-bit length.
Generating a key pair requires several steps:
Create a Key Pair Generator
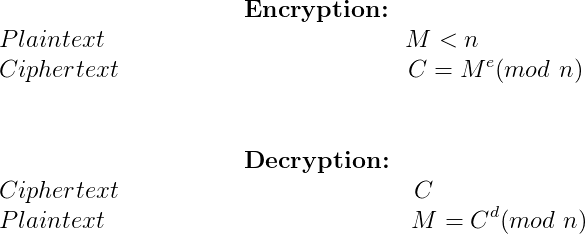
The first step is to get a key-pair generator object for generating keys for the DSA signature algorithm.
As with all engine classes, the way to get a KeyPairGenerator
object for a particular type of algorithm is to call the getInstance
static factory method on the KeyPairGenerator
class. This method has two forms, both of which hava a String algorithm
first argument; one form also has a String provider
second argument.
A caller may thus optionally specify the name of a provider, which will guarantee that the implementation of the algorithm requested is from the named provider. The sample code of this lesson always specifies the default SUN provider built into the JDK.
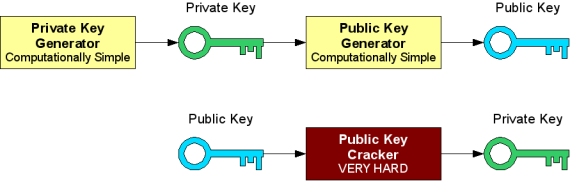
Put the following statement after the
line in the file created in the previous step, Prepare Initial Program Structure:
Initialize the Key Pair Generator
The next step is to initialize the key pair generator. All key pair generators share the concepts of a keysize and a source of randomness. The KeyPairGenerator
class has an initialize
method that takes these two types of arguments.
The keysize for a DSA key generator is the key length (in bits), which you will set to 1024.
The source of randomness must be an instance of the SecureRandom
class that provides a cryptographically strong random number generator (RNG). For more information about SecureRandom
, see the SecureRandom API Specification and the Java Cryptography Architecture Reference Guide .
The following example requests an instance of SecureRandom
that uses the SHA1PRNG algorithm, as provided by the built-in SUN provider. The example then passes this SecureRandom
instance to the key-pair generator initialization method.
Some situations require strong random values, such as when creating high-value and long-lived secrets like RSA public and private keys. To help guide applications in selecting a suitable strong SecureRandom
implementation, starting from JDK 8 Java distributions include a list of known strong SecureRandom
implementations in the securerandom.strongAlgorithms
property of the java.security.Security
class. When you are creating such data, you should consider using SecureRandom.getInstanceStrong()
, as it obtains an instance of the known strong algorithms.
Vb.net Public Private Key Generator Software
Generate the Pair of Keys
The final step is to generate the key pair and to store the keys in PrivateKey
and PublicKey
objects.
The access level of a declared element is the extent of the ability to access it, that is, what code has permission to read it or write to it. This is determined not only by how you declare the element itself, but also by the access level of the element's container. Code that cannot access a containing element cannot access any of its contained elements, even those declared as Public
. For example, a Public
variable in a Private
structure can be accessed from inside the class that contains the structure, but not from outside that class.
Public
The Public keyword in the declaration statement specifies that the element can be accessed from code anywhere in the same project, from other projects that reference the project, and from any assembly built from the project. The following code shows a sample Public
declaration:
Close PuTTYgen.Go to Start PuTTY Run PageantIt won’t really do anything at first. If the icon is not visible it may be hidden. Generate ssh key for filezilla mac. You will need to right-click on the little icon that appears in the bottom right system tray.
You can use Public
only at module, interface, or namespace level. This means you can declare a public element at the level of a source file or namespace, or inside an interface, module, class, or structure, but not in a procedure.
Protected
The Protected keyword in the declaration statement specifies that the element can be accessed only from within the same class, or from a class derived from this class. The following code shows a sample Protected
declaration:
You can use Protected
only at class level, and only when you declare a member of a class. This means you can declare a protected element in a class, but not at the level of a source file or namespace, or inside an interface, module, structure, or procedure.
Friend
Vb.net Public Private Key Generator For Bitcoin And Ethereum
The Friend keyword in the declaration statement specifies that the element can be accessed from within the same assembly, but not from outside the assembly. The following code shows a sample Friend
declaration:
You can use Friend
only at module, interface, or namespace level. This means you can declare a friend element at the level of a source file or namespace, or inside an interface, module, class, or structure, but not in a procedure.
Protected Friend
The Protected Friend keyword combination in the declaration statement specifies that the element can be accessed either from derived classes or from within the same assembly, or both. The following code shows a sample Protected Friend
declaration:
You can use Protected Friend
only at class level, and only when you declare a member of a class. This means you can declare a protected friend element in a class, but not at the level of a source file or namespace, or inside an interface, module, structure, or procedure.
Private
The Private keyword in the declaration statement specifies that the element can be accessed only from within the same module, class, or structure. The following code shows a sample Private
declaration:
You can use Private
only at module level. This means you can declare a private element inside a module, class, or structure, but not at the level of a source file or namespace, inside an interface, or in a procedure.
Public Private Key Encryption
At the module level, the Dim
statement without any access level keywords is equivalent to a Private
declaration. However, you might want to use the Private
keyword to make your code easier to read and interpret.
Private Protected
The Private Protected keyword combination in the declaration statement specifies that the element can be accessed only from within the same class, as well as from derived classes found in the same assembly as the containing class. The Private Protected
access modifier is supported starting with Visual Basic 15.5.
Vb.net Public Private Key Generator 2018
The following example shows a Private Protected
declaration:
You can declare a Private Protected
element only inside of a class. You cannot declare it within an interface or structure, nor can you declare it at the level of a source file or namespace, inside an interface or a structure, or in a procedure.
The Private Protected
access modifier is supported by Visual Basic 15.5 and later. To use it, you add the following element to your Visual Basic project (*.vbproj) file. As long as Visual Basic 15.5 or later is installed on your system, it lets you take advantage of all the language features supported by the latest version of the Visual Basic compiler:
To use the Private Protected
access modifier, you must add the following element to your Visual Basic project (*.vbproj) file:
For more information see setting the Visual Basic language version.
Access Modifiers
Vb.net Public Private Key Generator V2 4 Full Version
The keywords that specify access level are called access modifiers. The following table compares the access modifiers:
Vb.net Public Private Key Generator Blockchain
Access modifier | Access level granted | Elements you can declare with this access level | Declaration context within which you can use this modifier |
---|---|---|---|
Public | Unrestricted: Any code that can see a public element can access it | Interfaces Modules Classes Structures Structure members Procedures Properties Member variables Constants Enumerations Events External declarations Delegates | Source file Namespace Interface Module Class Structure |
Protected | Derivational: Code in the class that declares a protected element, or a class derived from it, can access the element | Interfaces Classes Structures Procedures Properties Member variables Constants Enumerations Events External declarations Delegates | Class |
Friend | Assembly: Code in the assembly that declares a friend element can access it | Interfaces Modules Classes Structures Structure members Procedures Properties Member variables Constants Enumerations Events External declarations Delegates | Source file Namespace Interface Module Class Structure |
Protected Friend | Union of Protected and Friend :Code in the same class or the same assembly as a protected friend element, or within any class derived from the element's class, can access it | Interfaces Classes Structures Procedures Properties Member variables Constants Enumerations Events External declarations Delegates | Class |
Private | Declaration context: Code in the type that declares a private element, including code within contained types, can access the element | Interfaces Classes Structures Structure members Procedures Properties Member variables Constants Enumerations Events External declarations Delegates | Module Class Structure |
Private Protected | Code in the class that declares a private protected element, or code in a derived class found in the same assembly as the bas class. | Interfaces Classes Structures Procedures Properties Member variables Constants Enumerations Events External declarations Delegates | Class |
See also
Related Articles
- Generate Ssh Key Linux Gitlab
- World In Conflict Soviet Assault Cd Key Generator
- Avatar The Game Key Generator
- Generate Ssh Key Gitlab Centos
- Norton Antivirus 2011 Product Key Generator Free
- Windows Server 2003 Enterprise Edition Product Key Generator
- Nfs Underground 2 Key Generator Download
- Wolfenstein 2 Steam Key Generator
- Wavepad Editor Key Generator Download
- 32 Bit Aes Key Generator